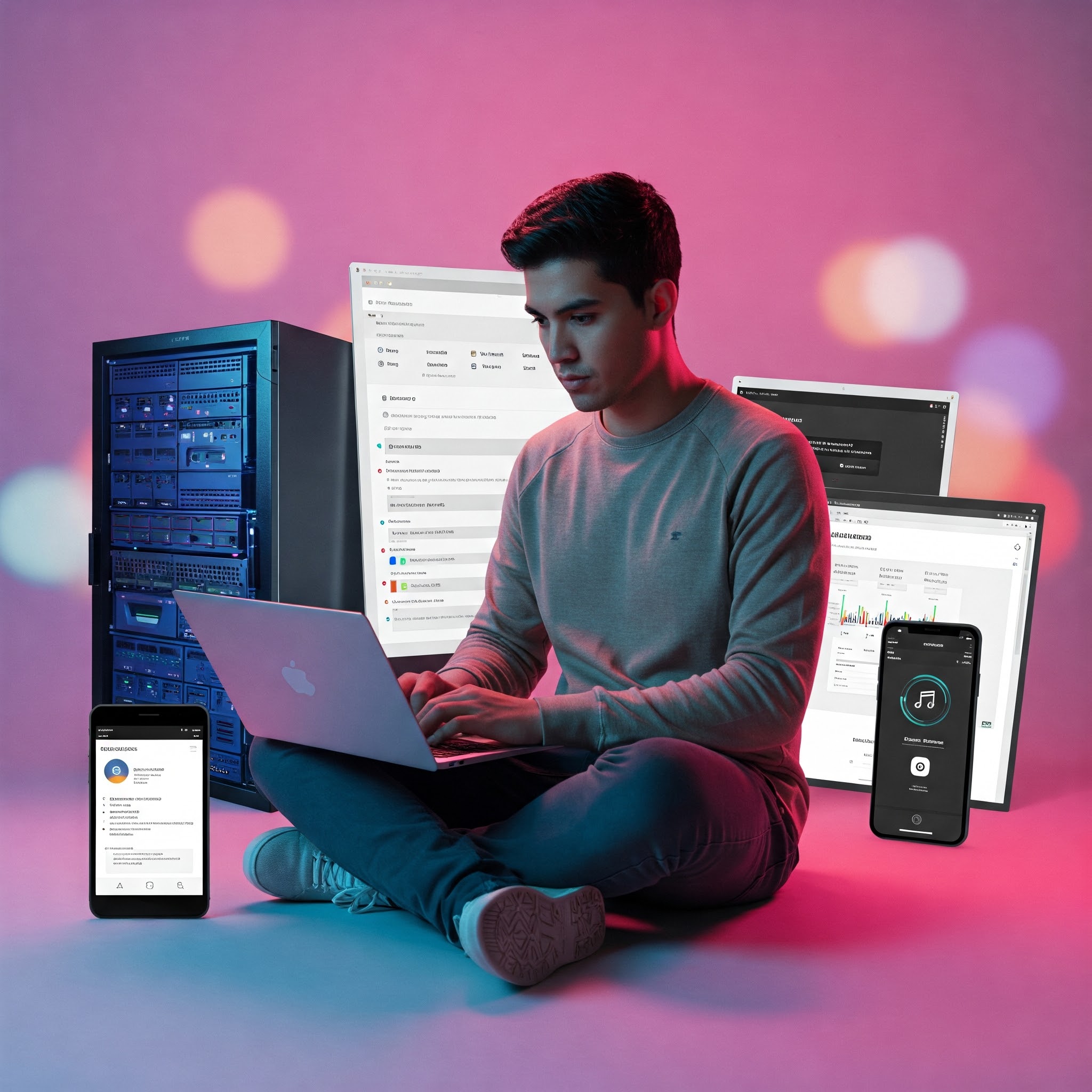
Transform Your Life with These 5 Self-Hosted Tools
In today's digital age, having the right tools can make a significant difference in your personal and professional life. This article explores five self-hosted tools that empower you to take control of your work, enhance productivity, and improve overall efficiency. From project management to data storage, discover how these tools can help you build a more organized and fulfilling lifestyle, all while ensuring your privacy and data security.